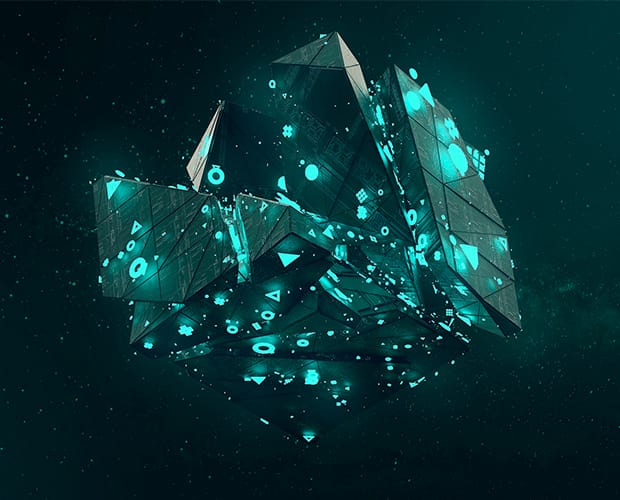
Python And PostgresSQL: Complete Guide
Best Seller
160 Lectures
21h 27m
Prepare for your Microsoft examination with our training course. The No_code course contains a complete batch of videos that will provide you with profound and thorough knowledge related to Microsoft certification exam. Pass the Microsoft No_code test with flying colors.
$13.99$24.99
Curriculum For This Course
- 1. Installing Python on Mac 1m
- 1. The Python interactive shell (IDLE) 4m
- 2. Numbers and Strings in Python 5m
- 3. Variables in Python 6m
- 4. The str() method to convert to Strings 4m
- 5. Running a Python file from the IDLE 7m
- 6. Getting user input in Python 6m
- 7. The int() method to convert to Integers 2m
- 8. The format() method to simplify String formatting 7m
- 1. Lists in Python 5m
- 2. The for loop in Python 3m
- 3. Boolean expressions, True and False 5m
- 4. If statements in Python 5m
- 5. The 'in' keyword to check whether a list contains an element 5m
- 6. Giving the user multiple chances in our program 9m
- 7. Generating random integers in Python 8m
- 8. Defining our own methods in Python 7m
- 9. Returning values from our methods in Python 5m
- 1. The String split() method 4m
- 2. List comprehension in Python 7m
- 3. Python Sets — what is a Set? 7m
- 4. Set comprehension in Python 6m
- 5. Creating our lottery numbers 8m
- 6. Calculating the lottery winnings 6m
- 1. Installing PyCharm, a professional Python tool 3m
- 2. Setting up PyCharm on Mac 2m
- 3. Setting up PyCharm on Windows 4m
- 4. Dictionaries in Python 9m
- 5. Advanced Dictionary usage in Python 8m
- 6. Methods returning dictionaries 7m
- 7. Appending to a list in Python 5m
- 8. Adding marks to our student data structure 9m
- 9. The sum() method in Python 8m
- 10. Iterating over a list and using the dictionaries inside it 6m
- 11. Creating the application menu 15m
- 1. What are classes? Classes in Python 12m
- 2. The Movie Class 8m
- 3. The User Class (and the __repr__ method) 6m
- 4. The filter() method in Python 10m
- 5. More Movie operations and methods 6m
- 6. Writing to a file in Python 4m
- 7. Saving CSV files with our data 10m
- 8. Loading our data from CSV files 12m
- 9. Saving JSON files with our data 6m
- 10. Loading our data from JSON files 8m
- 11. Argument unpacking in Python (the two asterisks) 6m
- 12. Creating the menu for our application 15m
- 1. Introduction to databases 13m
- 2. Installing PostgreSQL on Windows 4m
- 3. Using PostgreSQL on Windows 7m
- 4. Executing SQL queries on Windows 2m
- 5. Installing PostgreSQL on Mac 5m
- 6. Using PostgreSQL on Mac 9m
- 7. Executing SQL commands on Mac 1m
- 8. Using the sample data provided 3m
- 9. SQL: The SELECT command 8m
- 10. SQL: filtering with WHERE 4m
- 11. SQL: LIMIT for limiting the number of results 2m
- 12. SQL: UPDATE data in a table 6m
- 13. SQL: DELETE data from a table 5m
- 14. SQL Wildcards for filtering unknowns 5m
- 15. What is a JOIN? 14m
- 16. SQL: JOINs and JOIN examples 10m
- 17. SQL: GROUP BY for aggregation of data 9m
- 18. SQL: ORDER BY for sorting data 5m
- 19. SQL: CREATE TABLE 12m
- 20. SQL: INSERT INTO for adding data to a table 10m
- 21. SQL: SEQUENCE for auto-incrementing fields 7m
- 22. SQL: CREATE INDEX and advanced information about indexes 12m
- 23. SQL: DROP TABLE for deleting tables and data 6m
- 1. SQL: VIEWs and what they are used for 19m
- 2. SQL: built-in functions and the HAVING construct 12m
- 3. Dates in SQL: an old problem 14m
- 4. Other data types in SQL (including JSON in PostgreSQL) 11m
- 5. Nested SELECT statements for complex queries 9m
- 6. The PostgreSQL SERIAL type 3m
- 1. Installing psycopg2 on Windows (2018 update) 6m
- 2. Installing psycopg2 on Mac 4m
- 3. Verifying everything works—don't proceed if it doesn't! 3m
- 4. Mac OS X: Fixing psycopg2 'library not loaded' error 6m
- 5. What is a virtual environment? 8m
- 6. psycopg2 on a virtualenv on Windows (2018 update) 4m
- 7. Psycopg2 on a virtualenv on Windows (older versions) 5m
- 8. Setting up the app structure, pip, and requirements.txt 4m
- 9. Recap on classes and object-oriented programming 10m
- 10. Saving to database from Python 17m
- 11. Loading from the database from Python 16m
- 12. Removing some duplicate code from our app 4m
- 13. Connection pooling and why it is important 13m
- 14. Creating the ConnectionPool class 9m
- 15. Creating the ConnectionFromPool class 9m
- 16. Obtaining a cursor from the ConnectionFromPool class 8m
- 17. The Database class and selective initialisation 19m
- 18. Cleaning up the Database class 6m
- 19. End of section review 4m
- 1. What is an API? 26m
- 2. Making requests in Python 12m
- 3. What is OAuth? 7m
- 4. Creating a Twitter app 4m
- 5. Setting up Twitter login 5m
- 6. Getting the OAuth request token 15m
- 7. More on the Python debugger—an essential tool 5m
- 8. Getting authorization by the user 10m
- 9. Getting the OAuth access token 6m
- 10. Performing Twitter requests: getting images 17m
- 11. Reusing code from the last section to save users 11m
- 12. Creating users in our app 8m
- 13. Retrieving users in our app 14m
- 14. Cleaning up the code—extremely important! 19m
- 15. Introduction to Flask and Python Web Development 16m
- 16. Adding a Twitter login endpoint 11m
- 17. Adding Twitter authorization 23m
- 18. Creating the user profile 9m
- 19. The Flask before_request decorator 9m
- 20. Checking if a user is already logged in 3m
- 21. Searching tweets and displaying them 8m
- 22. Searching for different things 3m
- 23. What is Bootstrap? 9m
- 24. Writing our own CSS 6m
- 25. Allowing users to perform custom searches 7m
- 26. Adding sentiment analysis with another API 16m
- 1. What is Git? 5m
- 2. Installing Git on Mac and Windows 7m
- 3. Introduction to the UNIX terminal 8m
- 4. The VIM text editor, a powerful terminal editor 6m
- 5. Dealing with files in the UNIX terminal 4m
- 6. What is a Git repository? 6m
- 7. git init — create a Git repository 4m
- 8. git add and git commit — staging and committing 8m
- 9. git log — viewing past commits 2m
- 10. Creating a repository on GitHub 3m
- 11. git remote — managing remote repositories 3m
- 12. Adding your SSH key to GitHub 6m
- 13. What is a README file? Introduction to Markdown 7m
- 14. git pull — pulling other's changes 3m
- 1. OOP: Inheritance 15m
- 2. OOP: Multiple Inheritance in Python 5m
- 3. OOP: What is composition? 4m
- 4. OOP: What is encapsulation? 12m
- 5. Introduction to Exceptions in Python 11m
- 6. Creating our own Exceptions 7m
- 7. Some of the available built-in Exceptions 6m
- 8. Python built-in methods 21m
- 9. Assertions in Python 8m
- 10. Lambda expressions in Python 8m
- 11. More uses of lambda expressions 10m
- 12. Lambda expressions in the wild 17m
- 13. Introduction to unit testing with unittest 20m
- 1. What are data structures? 6m
- 2. What is a Linked List? 9m
- 3. Introduction to Linked List Assignment 10m
- 4. Programming our own Linked List in Python 9m
- 5. Creating a Queue 6m
- 6. Introduction to Queue Assignment 11m
- 7. Programming our own Queue in Python 15m
- 8. Creating a Stack 4m
- 9. Introduction to Stack Assignment 5m
- 10. Programming our own Stack in Python 7m
- 11. Introduction to Binary Tree Assignment 8m
- 12. Programming our own Binary Tree in Python 9m
Hot Exams
Isaca COBIT-2019 Exam Dumps
Microsoft PL-600 Exam Dumps
Microsoft SC-200 Exam Dumps
Microsoft MB-910 Exam Dumps
HashiCorp Terraform-Associate Exam Dumps
Test Prep LSAT-Test Exam Dumps
Microsoft AZ-204 Exam Dumps
Cisco 350-701 Exam Dumps
Cisco 350-401 Exam Dumps
Microsoft MS-900 Exam Dumps
Microsoft AZ-900 Exam Dumps
Salesforce Marketing-Cloud-Email-Specialist Exam Dumps
iSQI CTFL-AT Exam Dumps
Exin SIAMF Exam Dumps
PMI PMP Exam Dumps
ISC2 SSCP Exam Dumps
ISC2 CISSP Exam Dumps
Isaca CISA Exam Dumps
GIAC GCIH Exam Dumps
Exin EX0-105 Exam Dumps
PMI PMP Exam Dumps
Cisco 200-301 Exam Dumps
Microsoft AZ-104 Exam Dumps
Microsoft AZ-900 Exam Dumps
HashiCorp Terraform-Associate Exam Dumps
Isaca CISM Exam Dumps
Cisco 350-701 Exam Dumps
CompTIA SY0-701 Exam Dumps
Isaca CISA Exam Dumps
ISC2 CISSP Exam Dumps
ITIL ITIL-Practitioner Exam Dumps
Microsoft MS-900 Exam Dumps
Microsoft AZ-204 Exam Dumps
Test Prep LSAT-Test Exam Dumps
Microsoft SC-200 Exam Dumps
Linux Foundation CKS Exam Dumps
Isaca COBIT-2019 Exam Dumps
CompTIA CAS-004 Exam Dumps
CIPS L4M3 Exam Dumps
Microsoft PL-300 Exam Dumps
How to Open Test Engine .dumpsarena Files
Use FREE DumpsArena Test Engine player to open .dumpsarena files
Refund Policy

DumpsArena.com has a remarkable success record. We're confident of our products and provide a no hassle refund policy.

Your purchase with DumpsArena.com is safe and fast.
The DumpsArena.com website is protected by 256-bit SSL from Cloudflare, the leader in online security.